How to Check Array Empty or Not in PHP?
This tutorial provides a comprehensive guide on how to check whether an array is empty in PHP. It presents two approaches using the empty() function and the count() function, allowing developers to efficiently determine if an array contains any elements. By mastering these techniques, developers can handle empty arrays effectively in their PHP projects.
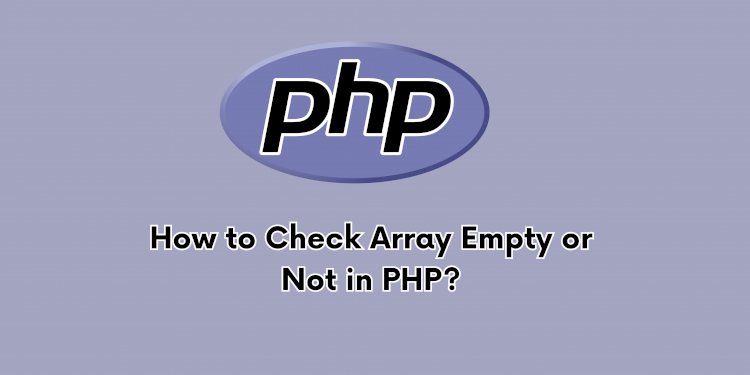
Today, we'll explore how to determine whether an array is empty or not in PHP. This tutorial offers step-by-step guidance, illustrating two different methods to check if an array is empty using PHP functions. By the end, you'll be equipped with the knowledge to efficiently handle empty arrays in your PHP projects.
Method 1: Using the empty()
Function
<?php
$array = [];
if (empty($array)) {
echo('The array is empty.');
} else {
echo('The array is not empty.');
}
?>
Output:
The array is empty.
Method 2: Using the count()
Function
<?php
$array = [];
if (count($array) === 0) {
echo('The array is empty.');
} else {
echo('The array is not empty.');
}
Output:
The array is empty.
These examples demonstrate how to effectively check whether an array is empty using PHP. Whether you prefer the simplicity of the empty()
function or the explicitness of comparing the count to zero, these methods ensure you can handle empty arrays with ease in your PHP applications.
I hope this tutorial proves helpful in your PHP endeavors!