How to Find Array Length in PHP?
Uncover the secrets of determining PHP array length with our comprehensive guide. Master the count() function, handle multidimensional arrays, and optimize performance. Learn how to apply these skills in PHP Laravel.
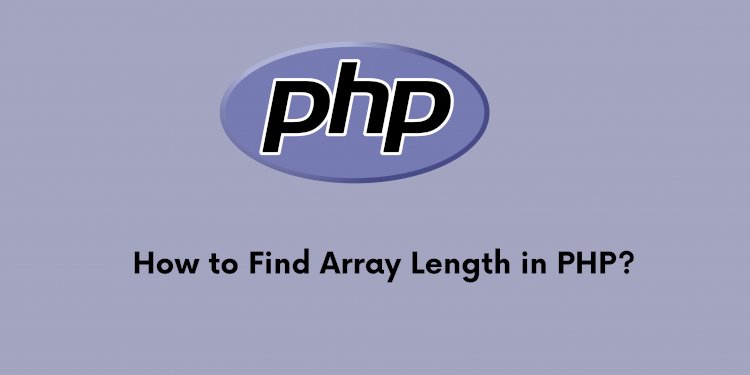
Introduction:
In this extensive tutorial, we will delve into the intricacies of determining the length of a PHP array. Understanding the size or length of an array is a fundamental operation in programming, and in this article, we will explore various aspects of achieving this in PHP. Whether you are a beginner seeking to learn the basics or a PHP developer aiming to enhance your knowledge, this guide will provide valuable insights. We will also touch upon how to apply these concepts in the context of PHP Laravel, a popular web application framework.
1. The Importance of Array Length:
- Explain why knowing the length of an array is crucial in programming.
- Discuss scenarios where array length is essential for data manipulation.
2. Basic Usage of `count()`:
- Introduce the `count()` function in PHP.
- Provide simple examples of using `count()` to find array length.
3. Handling Multidimensional Arrays:
- Explore how to determine the length of multidimensional arrays.
- Show how nested loops and `count()` can be used for this purpose.
4. Performance Considerations:
- Discuss the performance implications of using `count()` on large arrays.
- Offer alternative methods for getting array length in such cases.
5. Use Cases in PHP Laravel:
- Demonstrate practical examples of finding array length in Laravel applications.
- Highlight scenarios in web development where array length is valuable.
6. Alternative Techniques:
- Present other PHP functions and methods for calculating array length.
- Compare the pros and cons of different approaches.
7. Tips for Efficient Array Length Handling:
- Share best practices for optimizing array length retrieval in PHP.
- Address common mistakes and potential pitfalls.
8. Conclusion:
- Summarize the key takeaways from the tutorial.
- Emphasize the importance of mastering array length manipulation in PHP development.
By the end of this comprehensive guide, you will have a deep understanding of how to find the length of PHP arrays and how to apply this knowledge effectively in your web development projects, including those built with PHP Laravel.
<?php
$array = ['One', 'Two', 'Three', 'Four', 'Five'];
$length = count($array);
var_dump($length);
?>