How to Get Random Value from an Array in PHP?
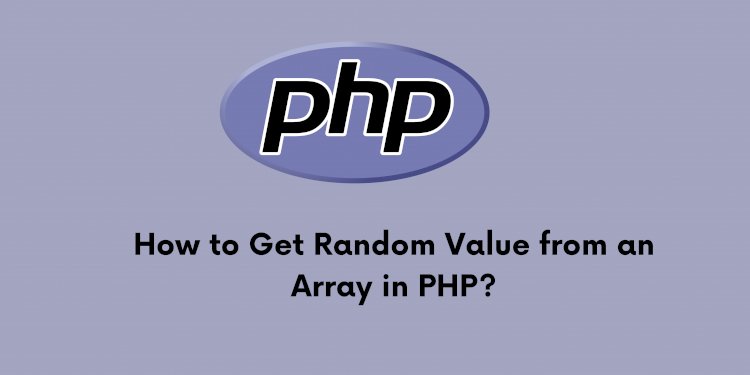
When working with PHP arrays, you might have the requirement to select a random value from an array. This guide demonstrates an efficient way to accomplish this task. Let's explore the steps for getting a random value from an array in PHP.
To retrieve a random value from a PHP array, you can use the array_rand()
function. Here's a straightforward example:
// Sample PHP array
$data = ["apple", "banana", "cherry", "date", "fig"];
// Use array_rand() to get a random array key
$randomKey = array_rand($data);
// Access the random value using the random key
$randomValue = $data[$randomKey];
// Display the random value
echo $randomValue;
In this example, we start with a PHP array named $data
, which contains various values. We employ the array_rand()
function to obtain a random key from the array. This function selects a random key from the array's keys.
Once we have the random key, we use it to access the corresponding value from the array, resulting in a random value.
The output will be a randomly selected value from the array, such as "banana," "cherry," or any other value present in the array.
By following this example, you can effectively retrieve a random value from a PHP array using the array_rand()
function.