How to Merge Two Array in PHP?
This article provides a comprehensive guide on how to merge two arrays in PHP using both the array_merge() function and the union operator (+). The tutorial includes step-by-step examples demonstrating each method, suitable for PHP developers who are working in various environments, including Laravel. The examples illustrate how to append arrays and merge multiple arrays effectively, showing the output for each method to help developers understand the implications of each approach.
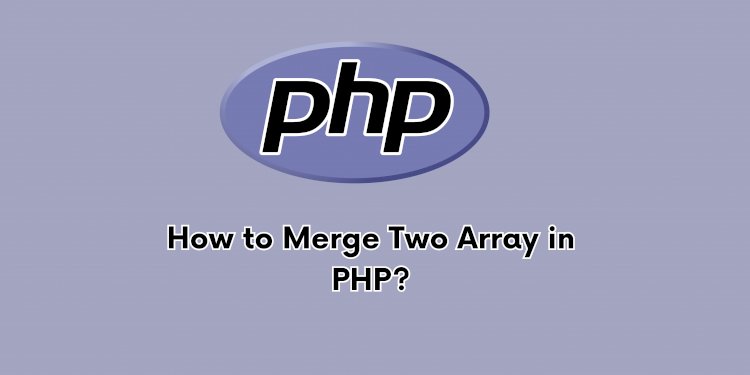
Hello Developer,
In this article, we'll explore how to merge two arrays in PHP, covering the use of the array_merge()
function and the union operator (+
). This guide will help you understand the differences and applications of each method when combining arrays in PHP, whether you're working in a Laravel environment or a plain PHP setup. We will look at how to append two arrays together and merge multiple arrays, with practical examples to illustrate each approach.
We'll use the array_merge()
function and the +
operator to demonstrate merging multiple arrays. Let's delve into the examples:
Example 1: Using array_merge()
<?php
$arrayOne = ["One", "Two", "Three"];
$arrayTwo = ["Four", "Five"];
$newArray = array_merge($arrayOne, $arrayTwo);
var_dump($newArray);
?>
Output:
array(5) {
[0]=> string(3) "One"
[1]=> string(3) "Two"
[2]=> string(5) "Three"
[3]=> string(4) "Four"
[4]=> string(4) "Five"
}
Example 2: Using the +
Operator
<?php
$arrayOne = [1 => "One", 2 => "Two", 3 => "Three"];
$arrayTwo = [4 => "Four", 5 => "Five"];
$newArray = $arrayOne + $arrayTwo;
var_dump($newArray);
?>
Output:
array(5) {
[1]=> string(3) "One"
[2]=> string(3) "Two"
[3]=> string(5) "Three"
[4]=> string(4) "Four"
[5]=> string(4) "Five"
}
This tutorial should help you effectively merge arrays in PHP, catering to different requirements and ensuring data integrity when combining array elements.