How to Remove String Values in PHP Array?
This article focuses on removing string values from a PHP array using the array_filter() function. It provides a simple example demonstrating how to remove string values from a PHP array. By following these steps, you'll learn how to effectively delete strings from an array in PHP. Let's dive into the process.
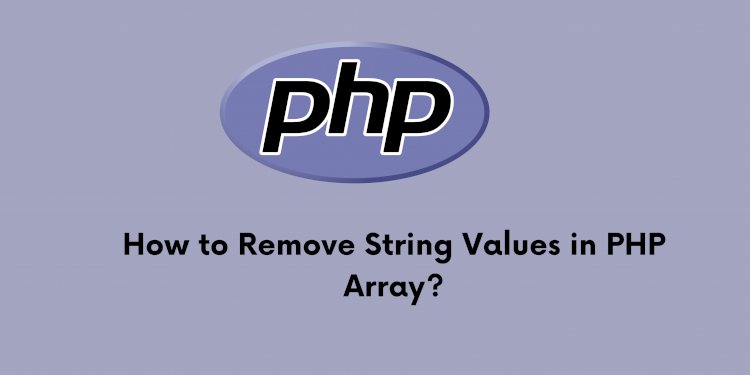
This article focuses on removing string values from a PHP array using the array_filter()
function. It provides a simple example demonstrating how to remove string values from a PHP array. By following these steps, you'll learn how to effectively delete strings from an array in PHP. Let's dive into the process.
We'll use the array_filter()
function to achieve this task. Here's a straightforward example of how to remove string values from a PHP array:
// Sample PHP array with mixed data types
$data = [42, 'apple', 56, 'banana', 'cherry'];
// Use array_filter() to remove string values
$result = array_filter($data, function ($value) {
return !is_string($value);
});
// Display the filtered array
print_r($result);
In this example, we start with a PHP array named $data
, which contains a mix of data types, including integers and strings. We then apply the array_filter()
function to filter out the string values. The anonymous function within array_filter()
checks if a value is not a string using !is_string($value)
and retains only non-string values.
The output will be a filtered array containing only non-string values:
Array
(
[0] => 42
[2] => 56
)