PHP Check If Array Has Duplicate Values
This PHP tutorial provides a comprehensive guide on detecting duplicate values within arrays. It demonstrates the usage of essential PHP functions like count() and array_unique() to efficiently identify and handle duplicate entries. By following this tutorial, developers gain valuable insights into array manipulation techniques, enhancing their proficiency in PHP programming.
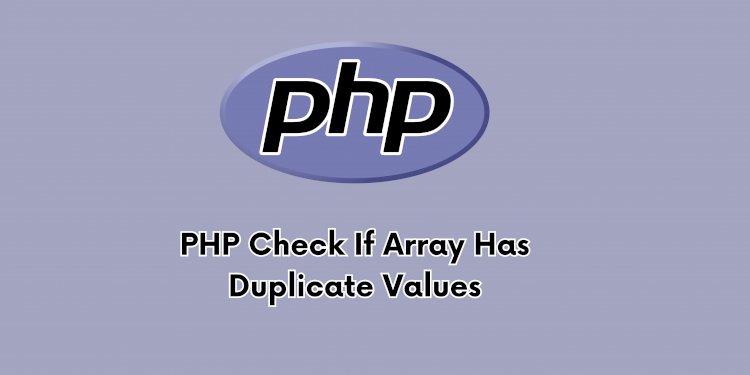
Today, we'll delve into the process of detecting duplicate values within a PHP array. By the end of this tutorial, you'll have a solid grasp of how to determine if an array contains duplicates, gaining insight into this fundamental PHP concept.
To begin, let's address the scenario where you need to ascertain whether your array holds duplicate values. This knowledge proves invaluable, especially when working on tasks that involve data validation or uniqueness checks.
We'll employ two key functions: count()
and array_unique()
, which play pivotal roles in our solution. The count()
function enables us to determine the total number of elements in an array, while array_unique()
efficiently filters out duplicate values, leaving us with a clean, unique array.
Without further ado, let's examine a simple yet effective code snippet that demonstrates how to perform this check:
index.php
<?php
$myArray = ['One', 'Two', 'Three', 'Two', 'Five', 'Three', 'One'];
if (count($myArray) !== count(array_unique($myArray))){
var_dump("Array has duplicate value.");
} else {
var_dump("Array does not have duplicate value.");
}
?>
Upon execution, this script meticulously examines our array and provides a clear verdict: whether duplicate values exist within it or not.
In the provided example, the output showcases the detection of duplicate values within the array, affirming our ability to identify such occurrences accurately.
By mastering this technique, you equip yourself with a powerful tool for ensuring data integrity and implementing robust algorithms in your PHP projects.
Stay tuned for more insights on PHP programming and array manipulation techniques!
Output:
string(26) "Array has duplicate value."