How to Get the First 10 Elements of an Array in PHP?
To retrieve the first 10 elements of a PHP array, you can use the array_slice() function
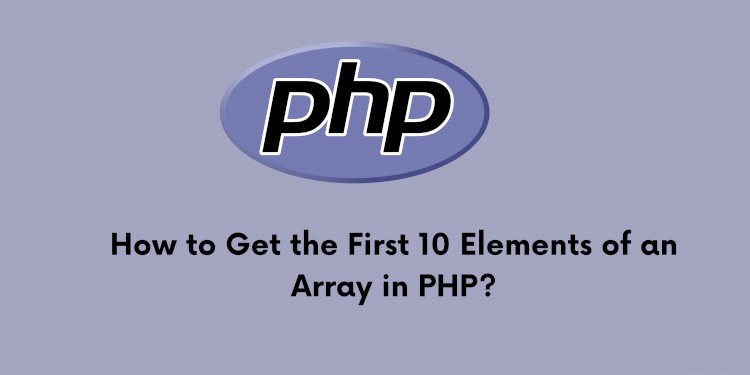
When working with PHP arrays, you might often need to extract the initial 10 elements from an array. This guide will illustrate how to accomplish this task efficiently in PHP. Let's explore the steps to achieve this.
To retrieve the first 10 elements of a PHP array, you can use the array_slice()
function. Here's a straightforward example:
// Sample PHP array
$data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12];
// Use array_slice() to extract the first 10 elements
$firstTen = array_slice($data, 0, 10);
// Display the first 10 elements
print_r($firstTen);
In this example, we begin with a PHP array named $data
, which contains a sequence of numbers. We employ the array_slice()
function to extract the first 10 elements from the array. The function takes three arguments: the input array, the starting index (0 in this case), and the length (10 in this case).
The output will be an array containing the first 10 elements:
Array
(
[0] => 1
[1] => 2
[2] => 3
[3] => 4
[4] => 5
[5] => 6
[6] => 7
[7] => 8
[8] => 9
[9] => 10
)