PHP Check If Array Has Duplicate Values
Learn how to identify duplicate values within a PHP array, ensuring data consistency and uniqueness in your code.
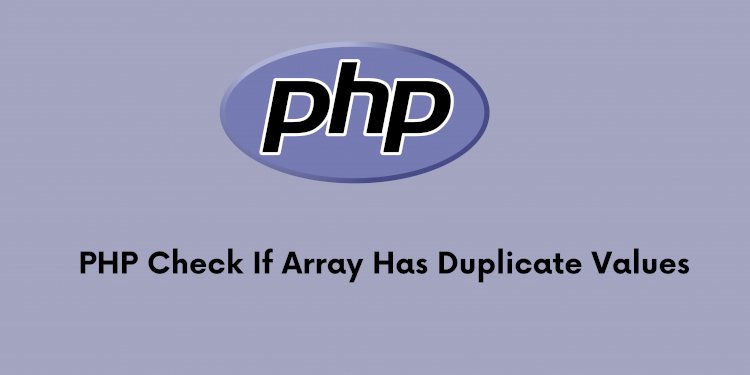
PHP Check If Array Has Duplicate Values
In this tutorial, I'll demonstrate how to check for duplicate values within a PHP array, helping you grasp the concept of identifying identical values within an array. You'll gain insights into determining whether PHP arrays share common values. If you're wondering how to check for duplicate values within a PHP Laravel array, I'll provide you with a straightforward example and solution.
We'll employ PHP's `count()` and `array_unique()` functions to efficiently check for duplicate values within an array. So, let's dive into the straightforward code that showcases how to identify duplicate values in a PHP array.
<?php
$myArray = ['One', 'Two', 'Three', 'Two', 'Five', 'Three', 'One'];
if (count($myArray) !== count(array_unique($myArray))) {
var_dump("The array contains duplicate values.");
} else {
var_dump("The array does not contain duplicate values.");
}
?>