How to Remove String Keys in PHP Array?
This concise tutorial demonstrates how to remove string keys from a PHP array using the array_filter() function along with is_numeric() and ARRAY_FILTER_USE_KEY. The provided code example illustrates the process effectively, ensuring that only numeric keys are retained in the resulting array.
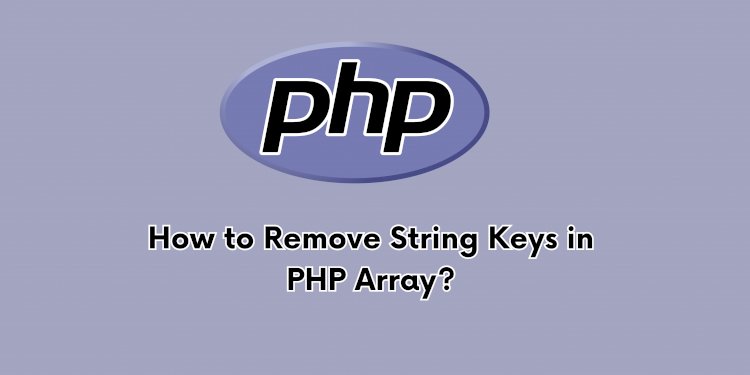
Welcome to this quick tutorial where we'll delve into removing string keys from a PHP array. We'll walk through a simple example to illustrate how to accomplish this task efficiently. By leveraging the array_filter()
function with is_numeric()
and ARRAY_FILTER_USE_KEY
, we'll effectively eliminate string keys from the PHP array. Let's dive into the code snippet:
<?php
$myArray = [1 => "One", "Two" => 2, 3 => "Three", "Four" => 4, 5 => "Five", "Six" => 6];
$newArray = array_filter(
$myArray,
function ($k) { return is_numeric($k); },
ARRAY_FILTER_USE_KEY
);
var_dump($newArray);
?>
Output:
array(3) {
[1]=> string(3) "One"
[3]=> string(5) "Three"
[5]=> string(4) "Five"
}
I hope this simple yet effective solution proves helpful in your PHP array manipulation tasks.