PHP : file_get_contents SSL: Connection Reset by Peer
Learn how to address the "file_get_contents SSL: Connection Reset by Peer" error in PHP when working with SSL connections. While disabling SSL verification is not recommended for production environments due to security risks, it may be necessary for debugging purposes. Utilize PHP's stream context to disable SSL verification temporarily, but exercise caution and prioritize security in production environments.
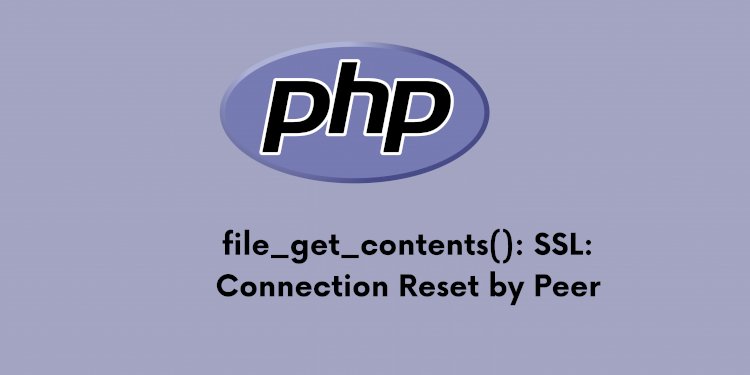
Firstly, it's important to note that disabling SSL verification in PHP is generally discouraged for production environments due to potential security vulnerabilities. However, in certain scenarios like debugging or specific tasks where security isn't a primary concern, you may need to disable SSL verification temporarily.
To achieve this, you can utilize PHP's stream context capabilities. Below is a snippet demonstrating how to disable SSL verification using stream_context_create
:
<?php
$context = stream_context_create([
'ssl' => [
'verify_peer' => false,
'verify_peer_name' => false,
],
]);
$content = file_get_contents('https://example.com', false, $context);
Here's a breakdown of what's happening in the code:
- We create a stream context using
stream_context_create
. - Inside this context, we set the
verify_peer
andverify_peer_name
options tofalse
, effectively turning off SSL certificate verification.
However, it's crucial to reiterate that this approach should only be used sparingly and with caution. Disabling SSL verification can potentially expose your application to security risks. It's advisable to address the underlying SSL issue or employ trusted SSL certificates to ensure a secure connection.
I hope this explanation helps clarify the issue and provides a viable solution for your PHP SSL-related challenges.