How to Check Key Exists in Array in PHP?
This tutorial provides a detailed explanation on how to check for the presence of keys in PHP arrays. Using straightforward examples, the article demonstrates two commonly used PHP functions.
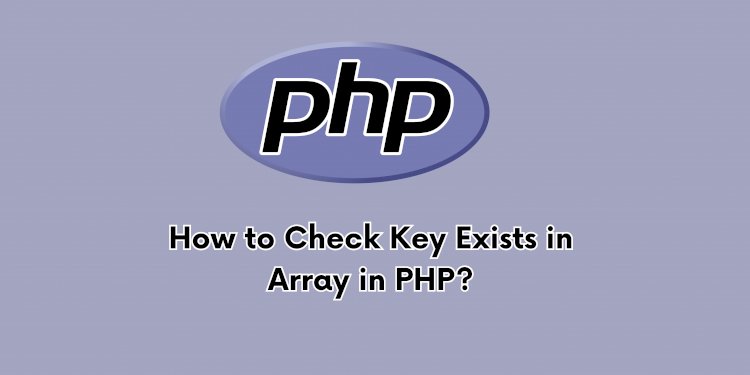
Hey Developer,
Welcome to this guide on verifying the presence of keys within arrays in PHP. This tutorial will take you step-by-step through the process, ensuring you understand how to check if a key is present in an array. By the end, you'll have learned two different methods to determine if an array key is set or defined.
We'll be using the array_key_exists()
function and the isset()
function to check if a key exists in a PHP array. Let's go through some simple code examples to illustrate these methods.
Example 1: Using array_key_exists()
<?php
$array = ['id' => 1, 'name' => 'Modi', 'email' => '[email protected]'];
if (array_key_exists('name', $array)) {
echo('The key exists.');
} else {
echo('The key does not exist.');
}
?>
Output:
The key exists.
Example 2: Using isset()
<?php
$array = ['id' => 1, 'name' => 'modi', 'email' => '[email protected]'];
if (isset($array['name'])) {
echo('The key exists.');
} else {
echo('The key does not exist.');
}
?>
Output:
The key exists.
These examples demonstrate how to effectively check for the existence of a key in a PHP array. Whether you're using array_key_exists()
to explicitly check for keys, or isset()
to verify if the key is not only present but also not null, these tools are invaluable in PHP development.
I hope this tutorial helps you in your PHP projects. Happy coding!